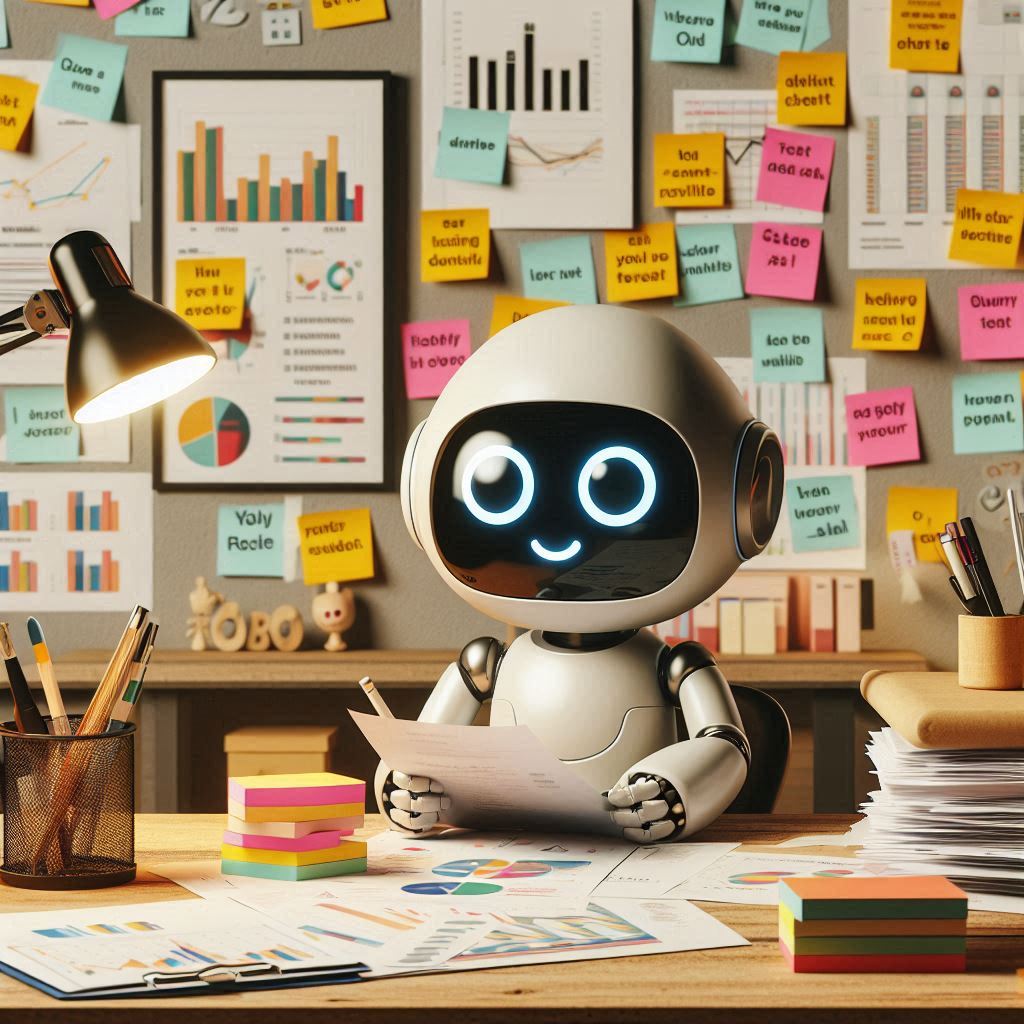
If you want to have more information on AI Endpoints, please read the following blog post.
You can, also, have a look at our previous blog posts on how use AI Endpoints.
OVHcloud AI Endpoints allows developers to easily add AI features to there day to day developments.
In this blog post, we will explore how to create a sentiments analyzer using OVHcloud AI Endpoints.
OVHcloud AI Endpoints provides a lot of models, but for this example we will use models from the Natural Language Processing (NLP) family.
The bellow demonstration will use roberta-base-go_emotions model.
Let’s create this analyzer with Java.
ℹ️ You can find the full code on Github ℹ️
Add the dependencies to the pom.xml
To make the code simpler you will use Quarkus.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ovhcloud.examples.aiendpoints</groupId>
<artifactId>nlp</artifactId>
<version>1.0.0-SNAPSHOT</version>
<name>nlp</name>
<url>https://endpoints.ai.cloud.ovh.net/</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<compiler-plugin.version>3.12.1</compiler-plugin.version>
<maven.compiler.source>21</maven.compiler.source>
<maven.compiler.target>21</maven.compiler.target>
<maven.compiler.release>21</maven.compiler.release>
<quarkus.platform.artifact-id>quarkus-bom</quarkus.platform.artifact-id>
<quarkus.platform.group-id>io.quarkus.platform</quarkus.platform.group-id>
<quarkus.platform.version>3.11.0</quarkus.platform.version>
<skipITs>true</skipITs>
<surefire-plugin.version>3.2.5</surefire-plugin.version>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>${quarkus.platform.group-id}</groupId>
<artifactId>${quarkus.platform.artifact-id}</artifactId>
<version>${quarkus.platform.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-arc</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-rest</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-rest-client-jackson</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-junit5</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<!-- ... -->
</project>
ℹ️ The full pom.xml is available here.
Create the service to call AI Endpoints
To do this, we use Eclipse MicroProfile and Jakarta EE.
package com.ovhcloud.examples.aiendpoints.nlp.sentiment;
import org.eclipse.microprofile.rest.client.annotation.ClientHeaderParam;
import org.eclipse.microprofile.rest.client.inject.RegisterRestClient;
import jakarta.ws.rs.POST;
import jakarta.ws.rs.Path;
@Path("/api")
@RegisterRestClient(baseUri = "https://roberta-base-go-emotions.endpoints.kepler.ai.cloud.ovh.net")
@ClientHeaderParam(name = "Authorization", value = "Bearer ${ovhcloud.ai-endpoints.token}")
@ClientHeaderParam(name = "Content-Type", value = "application/json")
public interface AISentimentService {
@POST
@Path("text2emotions")
EmotionEvaluation text2emotions(String text);
}
ℹ️ You will find the EmotionEvaluation source code here.
Use the service to analyze text
To do this, we use, again, Eclipse MicroProfile and Jakarta EE.
At the end, we expose a REST endpoint that allows us to do text sentiments analysis.
package com.ovhcloud.examples.aiendpoints.nlp.sentiment;
import org.eclipse.microprofile.rest.client.inject.RestClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import jakarta.ws.rs.POST;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.MediaType;
/**
* Class to do textual sentiments analysis.
*/
@Path("/sentiment")
public class SentimentsAnalysisResource {
private static final Logger _LOG = LoggerFactory.getLogger(SentimentsAnalysisResource.class);
@RestClient
private AISentimentService aiSentimentService;
@POST
@Produces(MediaType.TEXT_PLAIN)
public String getSentiment(String text) {
_LOG.info("Sentiment analysis for text: {}", text);
return aiSentimentService.text2emotions(text).toEmoji();
}
}
Now it’s time to test the code!
Start Quarkus in dev mode: quarkus dev
To do this, simply do a POST request to the http://localhost:8080/sentiment URL with a text as payload.
Don’t hesitate to test our new product, AI Endpoints, and give us your feedback.
You have a dedicated Discord channel (#ai-endpoints) on our Discord server (https://discord.gg/ovhcloud), see you there!
Once a developer, always a developer!
Java developer for many years, I have the joy of knowing JDK 1.1, JEE, Struts, ... and now Spring, Quarkus, (core, boot, batch), Angular, Groovy, Golang, ...
For more than ten years I was a Software Architect, a job that allowed me to face many problems inherent to the complex information systems in large groups.
I also had other lives, notably in automation and delivery with the implementation of CI/CD chains based on Jenkins pipelines.
I particularly like sharing and relationships with developers and I became a Developer Relation at OVHcloud.
This new adventure allows me to continue to use technologies that I like such as Kubernetes or AI for example but also to continue to learn and discover a lot of new things.
All the while keeping in mind one of my main motivations as a Developer Relation: making developers happy.
Always sharing, I am the co-creator of the TADx Meetup in Tours, allowing discovery and sharing around different tech topics.